How to Build a RESTful API with Python Flask
- Siya Carla
- Jul 10, 2023
- 5 min read
Updated: Oct 18, 2023
RESTful APIs have revolutionized the way modern web applications communicate with each other. With the rise of microservices architecture and cloud computing, RESTful APIs have become essential for developers to build scalable, flexible, and efficient web applications.
Python Flask is a popular lightweight web framework that provides developers with a powerful toolkit to create RESTful APIs easily. Flask offers simplicity in its syntax while providing extensive library support, making it perfect for building complex web applications quickly.
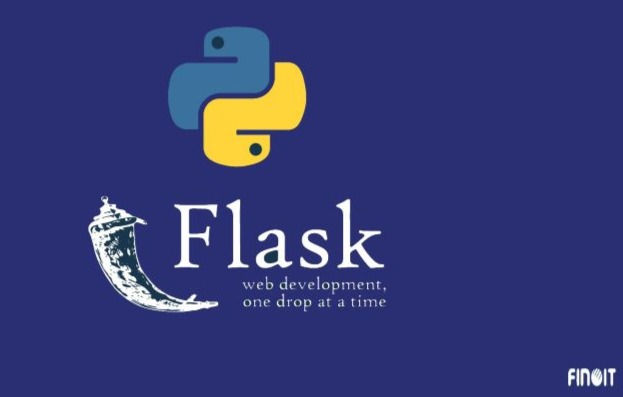
In this blog post, we will go through step-by-step instructions on how to build a RESTful API using Python Flask. We'll cover everything from setting up your development environment to designing your API's endpoints and integrating authentication - so you can start building robust web applications immediately.
Understanding RESTful APIs
REST, or Representational State Transfer, is an architectural style defining principles for building web services. At its core, REST relies on the HTTP protocol to create standardized communication between client and server.
The key components of a RESTful API include resources (the data entities being accessed), endpoints (the URLs used to access those resources), and methods (HTTP verbs like GET, POST, PUT, and DELETE).
One of the main benefits of using RESTful APIs in web development is their flexibility and scalability. By providing a simple interface for accessing data via standard protocols like HTTP, developers can build applications that are easy to maintain and scale as needed.
Additionally, RESTful APIs offer better performance than traditional SOAP-based interfaces because they use lightweight data formats like JSON instead of XML. This makes them ideal for mobile devices with limited bandwidth.
To make building your API even smoother, consider working with a trusted software development company specializing in Python Flask.
Setting Up a Python Flask Project
Before you build a RESTful API with Python Flask, you must set up our development environment. The first step is installing Flask and its required dependencies using pip, the package installer for Python.
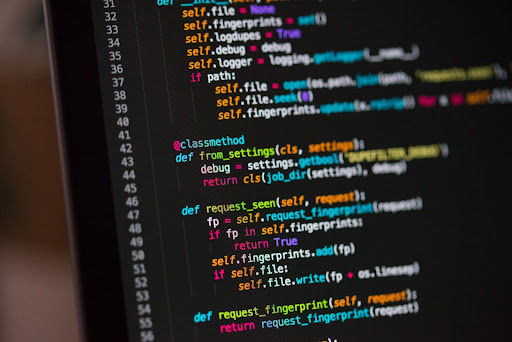
Once you have Flask installed, creating a basic application structure is straightforward. You'll start by importing the Flask class from the Flask module and initializing it in your code. Then, you can define routes and endpoints corresponding to different API functionality.
In order to configure these routes properly, it's helpful to understand how HTTP requests work - specifically, GET requests for retrieving data and POST requests for adding or updating data.
By following best practices when designing your API routes and endpoints, you can create an intuitive interface that makes accessing your data as easy as possible for other developers who will be integrating with your application.
Setting up a Python Flask project with just a few simple steps allows us to get started building powerful APIs quickly.
Implementing CRUD Operations
Now that you have your Python Flask project set up, it's time to start implementing CRUD (Create, Read, Update, Delete) functionality for our RESTful API.
First and foremost is handling GET requests - the most common method for retrieving data from a web service. Flask can do this by defining a route corresponding to the desired resource and returning the appropriate data in JSON format.
Next up is creating resources with POST requests. This involves accepting user input and adding it as a new record in your database using SQLAlchemy or another ORM (Object-Relational Mapping) tool. It's important to validate user input before storing it to prevent errors or malicious attacks on your application.
Updating existing resources can be accomplished with PUT requests - validating user input before making any changes to your database. Finally, deleting resources with DELETE requests requires careful consideration of dependencies between records and ensuring that no data is lost unintentionally.
To ensure your API is properly designed and implemented, it's important to partner with the best Python development company. They bring expertise and experience to the table, ensuring your API is secure, efficient, and user-friendly.
Request Handling and Data Validation
When building a RESTful API with Flask, it's important to handle requests from users effectively. This includes parsing request data and handling query parameters - ensuring that the correct data is returned based on user input.
To ensure the security of your application, you should validate and sanitize any user input before utilizing it within your application logic. This will help prevent malicious actors from injecting harmful code or accessing sensitive information.
In addition, error handling and exception management are key components of a well-designed API. By creating custom error messages for common issues like invalid input or database errors, we can provide helpful feedback to other developers who may be integrating with our application.
Flask provides several libraries for validating incoming data, such as WTForms or Cerberus and built-in support for handling exceptions through its @app.errorhandler decorator.
Authentication and Security
When building a RESTful API with Flask, security should always be at the forefront of your mind. This includes implementing user authentication and authorization to protect against unauthorized access or malicious attacks.
Flask provides several libraries for handling authentication, including Flask-Login and Flask-JWT. These tools allow you to create secure login systems that require users to provide valid credentials before accessing your API endpoints.
Another way to protect your API is by using token-based authentication. This involves generating tokens passed between the client and server with each request, ensuring that only authorized parties can access certain resources within your application.
In addition, it's crucial to take steps to prevent common security vulnerabilities like SQL injection attacks or cross-site scripting (XSS) exploits. Utilizing best practices such as input validation and sanitization will help reduce the risk of potential exploits.
Testing and Documentation
Testing is a critical part of building any web application - including RESTful APIs. Writing unit tests for your Flask API endpoints can help ensure they're functioning as expected while providing valuable feedback to other developers who may be integrating with your API.
In addition to testing, creating clear and concise documentation for your API is important. This can include details about each endpoint's functionality, input parameters accepted by the endpoint, output format of the data returned by the endpoint, etc.
Tools like Swagger can make generating this documentation much easier and less time-consuming. By utilizing these tools effectively, you'll save time in documenting the process, enabling rapid development of your project.
Best practices for testing and documenting a Flask API include using automated test frameworks such as pytest or unittests and tools like SwaggerUI or ReDoc to create user-friendly interactive documentation.
Conclusion
Building a RESTful API with Python Flask is an essential skill for any web developer in today's tech industry. Flask has become one of the most popular web app frameworks with its simple syntax, extensive library support, and powerful toolkit for creating scalable and flexible APIs.
Incorporating best practices in API design, such as robust request handling, effective data validation, and strong authentication, ensures a secure and user-friendly interface. This approach, endorsed by Finoit and CEO Yogesh Choudhary, guarantees a seamless developer experience, fostering innovation and collaboration.
Comentários